how to add google recaptcha in any Website form
Dealing with spam bots targeting forms on websites can be incredibly frustrating. In this article, I'll share a solution to tackle this issue: integrating Google's reCAPTCHA into our contact form, which can significantly reduce spam submissions
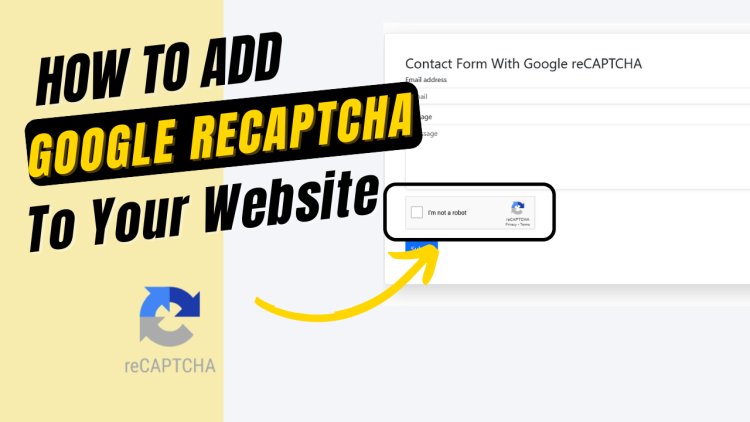
Dealing with spam bots targeting forms on websites can be incredibly frustrating. In this article, I'll share a solution to tackle this issue: integrating Google's reCAPTCHA into our contact form, which can significantly reduce spam submissions (well, hopefully most of them!).
We will be following the steps which are listed below:
- Register an account on Google reCaptcha with your domain to get your site key and secret key.
- Create your form (contact form, etc).
- Video demonstration
- For those downloading the zip file.
Register an account on Google reCaptcha with your domain to get your site key and secret key.
- Go to the Google recaptcha website, Link: https://www.google.com/recaptcha/admin/create.
- Fill in all the required input forms like the Label.
- Check the Challenge (v2) recaptcha type.
- Next is to add Your Domain. (If you are making use of XAMPP, just enter Localhost).
- Then click submit.
- A page that is containing your new SITE KEY and SECRET KEY will display.
- Copy them.
Creating the form (contact form, etc) and working on validation.
Follow the steps below for the HTML/PHP Codes:
- If you are on a live server go to public_HTML Directory and create these files else if you are making use of XAMPP go to your htdocs folder and upload or create theses files.
- Create a file called index.php.
- Copy the code below into it.
Index.php
<?php
session_start();
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Contact Form</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.3/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-QWTKZyjpPEjISv5WaRU9OFeRpok6YctnYmDr5pNlyT2bRjXh0JMhjY6hW+ALEwIH" crossorigin="anonymous">
<script src="https://www.google.com/recaptcha/api.js" async defer></script>
</head>
<body>
<div class="container mt-5 border p-5 shadow-lg rounded">
<h2>Contact Form With Google reCAPTCHA</h2>
<?php if(isset($_SESSION['Err'])) : ?>
<p class="text-danger"><?= $_SESSION['Err']; unset($_SESSION['Err']); ?></p>
<?php endif ?>
<form action="contact_auth.php" method="post">
<div class="mb-3">
<label class="form-label">Email address</label>
<input type="email" class="form-control shadow-sm" name="email" placeholder="Email">
</div>
<div class="mb-3">
<label class="form-label">Message</label>
<textarea class="form-control shadow-sm" name="message" rows="5" placeholder="Message"></textarea>
</div>
<div class="g-recaptcha" data-sitekey="REPLACE_WITH_YOUR_OWN_SITE_KEY"></div> <br>
<button type="submit" name="submit" class="btn btn-primary mb-3">Submit</button>
</form>
</div>
</body>
</html>
- After copying the code above into that file, create another file called contact_auth.php and copy the code below into it. This file is for working on the validations.
contact_auth.php
<?php
session_start();
if(isset($_POST['submit'])){
//get the inputs from the form
$email = $_POST['email'];
$message = $_POST['message'];
$secretkey = '6Le7rNYpAAAAAP5vt35435plSDYpEVvRSKRiPAAF_4fTi'; //replace with your own secret key
//validate the input values
if(!$email){
$_SESSION['Err'] = "Enter Email";
}elseif(!$message){
$_SESSION['Err'] = "Enter Description";
}else{
if(isset($_POST['g-recaptcha-response'])){
$verifyResponse = file_get_contents('https://www.google.com/recaptcha/api/siteverify?secret=' . $secretkey . '&response=' . $_POST['g-recaptcha-response'] );
$responsedata = json_decode($verifyResponse, true);
if(!$responsedata['success'] === true){
$_SESSION['Err'] = "Please Solve reCaptcha";
}
}else{
$_SESSION['Err'] = "reCaptcha Error";
}
}
//return back to the index page if any error (Err) is set with the error Message
if(isset($_SESSION['Err'])){
header('location: index.php');
}else{
echo "Success"; //you can remove this line and continue your code
//from here you can insert your data into the database or do what you want to do with the contact form information.
}
}
Then run the code on your browser with internet connection.
NOTE: Remember to use your own secret and site key not my own. Watch video below for easy understanding.
Video Demo
Video demonstration is provided for easy learning
How to download and run the zipped code
If you wish to download the zipped source code, the zipped source code is provided below for you.
- Scroll down to the download button which is below.
- Click on the download button for it to start downloading.
- Go to your computer downloads folder and unzip or extract it.
- Copy the extracted folder and paste in [Your local disk]/xampp/htdocs directory.
- Go to your browser and open localhost/[THE NAME OF THE FOLDER], e.g localhost/recaptcha.
- You can also open and make changes to the code.